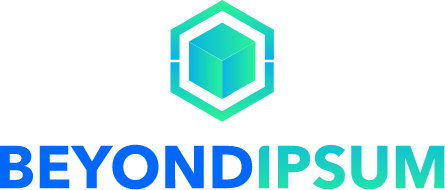
Unlock the Power
of Blockchain with BeyondIpsum
Your Trusted Partner for Node as a Service, DeFi Consulting, and Custom Solutions
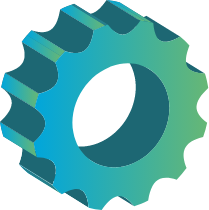
Nodes as a Service
At BeyondIpsum, we provide reliable and secure blockchain nodes as a service, tailored to meet the needs of both individuals and DeFi projects. Our nodes ensure fast and uninterrupted access to the blockchain, allowing you to focus on what matters most—building and growing your projects.
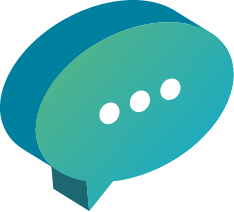
DeFi Project Consulting
Leverage our deep expertise in DeFi to navigate the complexities of decentralized finance. Whether you're launching a new project or looking to optimize your existing strategies, our consulting services offer the insights and guidance you need to succeed in the fast-paced world of blockchain.
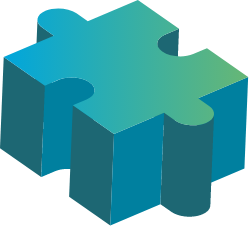
Data & Analytics Solutions
BeyondIpsum specializes in crafting custom tools and backend solutions tailored to your specific needs. Whether you require advanced data gathering, analytics, or API integration, we develop bespoke solutions that leverage the full power of our blockchain nodes, allowing you to focus on contract creation and front-end development.
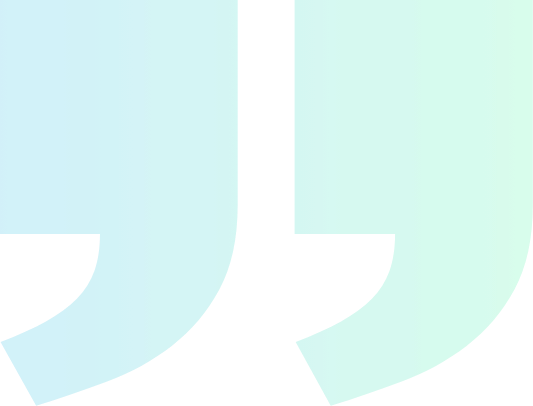